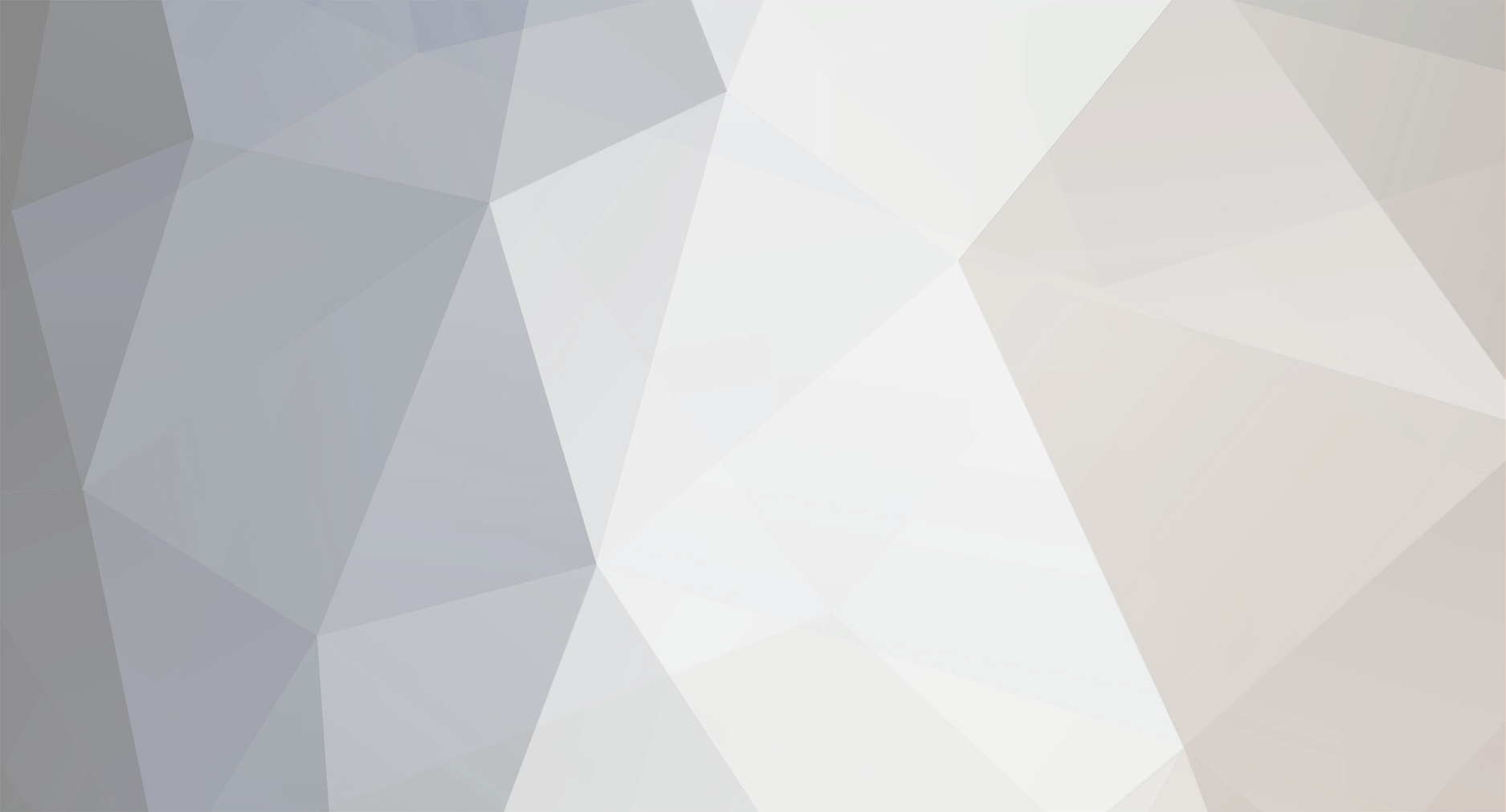
abdus
Members-
Posts
743 -
Joined
-
Last visited
-
Days Won
42
Everything posted by abdus
-
Yeah, this is the normal behavior. It's like any other page. Unless FormBuilder supports paginated forms, all fields will be listed vertically like this which will give you a very long page. And the browser will have no choice but to show the scrollbar.
-
Using Database class is actually quite straightforward. It's nothing but a wrapper around PHP's PDO class with some PW specific features. You prepare a SQL statement using prepare() method, then bind input values, execute() it and fetch() the results. <?php namespace ProcessWire; // sanitization $tableName = inputPost()->text('table'); $columnName = inputPost()->text('column'); $id = inputPost()->int('id'); // this is where you'd sanitize user input to prevent SQL injection $table = database()->escapeTable($tableName); $column = database()->escapeCol($columnName); $q = database()->prepare("SELECT `$column` FROM `$table` WHERE id = :id"); $q->bindValue(':id', $id); // always bind values instead of concatenation // $success = false; $values = null; try { $success = $q->execute(); $values = $q->fetchAll(); } catch (\Exception $e) { wire()->log($e->getMessage(), Notice::log); } if (!$success) { // handle error } if ($values) { // use values } To see how PW utilizes this class, check out Fieldtype.php, FieldtypeMulti.php, PagesEditor.php and PagesLoader.php from the core.
-
Because the form is too long to fit the viewport, so the browser shows the scrollbar? Do you see something else?
-
I know, but this error points out that some ini file somewhere is trying to load the php_soap extension from an nonexistent path. To find if there's a call to soap methods, you can perform a project wide search using the methods here http://php.net/manual/en/book.soap.php
-
Find your php.ini and comment out this line ;extension=php_soap.dll To find php.ini you can use echo php_ini_loaded_file(); http://php.net/manual/en/function.php-ini-loaded-file.php
-
Like @Soma said, I guess this is for preventing circular references when using the field in selectors. It's better if you add it manually in your code
-
Hmm, you can forcibly add it using this hook, though, (not tested) // site/ready.php wire()->addHookAfter('InputfieldPage::getSelectablePages', function (HookEvent $e) { $e->return->add($e->arguments(0)); });
-
It turns out PW doesn't allow that. /** * Return PageArray of selectable pages for this input * * @param Page $page The Page being edited * @return PageArray|null * */ public function ___getSelectablePages(Page $page) { // ... if($children && $children->has($page)) { $children->remove($page); // don't allow page being edited to be selected } return $children; }
-
this seems like the best approach. If these parents have a distinctive feature that can be used to select them (a certain parent, template, field etc), then use $pages->getIDs() method and update your selector to exclude the children with $pages("..., has_parent!=$hidableParents"); Edit: has_parent supports single values, you can build a string for multiple OR groups (has_parent=!35), (has_parent=!72), (...) etc
-
@benbyf, What you need is an inputfield that extends InputfieldTextarea and implements at least render() and processInput() methods. Using some JS and a process module you can let user select a folder and save it to the textarea. Start by creating a class that extends InputfieldTextarea, and add a button that fires up a modal to an intermediate process module. You use this module to show a list of folders that user can select using DropboxAPI. then when you get the input from the modal (check out JavaScript files for pwimage and pwlink CKEditor plugins, and ProcessPageEditImageSelect and ProcessPageLinkSelect modules), you save it to a (hidden) textarea (that you rendered in render() method). Using processInput() method you then validate the folder path, clean it up and send it to PW to save on the DB.
-
To live on the bleeding edge
-
It only stores path and metadata for the files. Actual files go to site/assets/files folder. Migration is quite easy, just dump the database, import into remote server, update database credentials in config.php, upload files, check file permissions, and done. Or use Site Profile Exporter module
-
Really cool project. I am used to developing individual sites that with separate wire directories but it gets tedious to update each and every one of them, and not to mention duplicate /wire/ directories. I was thinking of moving to the official multi-site solution, but I'll start with your module. Thanks for sharing it with us. Although for simple configurations this may not matter, I've found out that using PHP files for configuration files are much easier to handle, and doesn't need parsing at all. It's as simple as this: <?php // mysite.config.php return [ 'site' => 'mysite', 'domain' => 'mysite.com' ]; <?php // ConfigReader.php $configs = []; foreach(configFiles in configDir) { $configs[] = include(configFile); } https://github.com/mrkrstphr/array-config To export as php configuration files you can use http://php.net/manual/en/function.var-export.php Major disadvantage is that it's php only whereas JSON is almost universal.
-
@suntrop, I've updated my hook to include sorting by labels wire()->addHookAfter('ProcessPageEdit::buildFormSettings', function (HookEvent $e) { $page = $e->object->getPage(); if ($page->template != "my-template") return; $templatesField = $e->return->children->get('id=template'); $templates = $templatesField->getOptions(); // sort first asort($templates); foreach ($templates as $tid => $tname) { // remove incompatible fields $template = $e->templates->get($tid); if (!$template) continue; if (strpos($template->tags, 'my-template-compatible') === false) unset($templates[$tid]); } $templatesField->set('options', $templates); });
-
Update your graphics driver, try rolling back to an older version of chrome
-
Starting with the modules or plugins, despite being productive initially, causes more issues in the long run. This is also, I think, the major disadvantage of WordPress. Majority of the backend is inaccessible to beginners, and the core is obnoxiously complicated. How can showing some posts be so difficult (you know, "the loop"). This forces you to use plugins over plugins and the only thing you learn in the process is how to prevent them from spontaneously disintegrate. I agree with what @Peter Knight said. Even though there are modules for most scenarios, I enjoy recreating those modules by hand, because I get to practice coding and explore new/better/faster approaches, and I also get familiar with the inner workings of ProcessWire. Maybe in the future I'll get bored of building things from scratch but then I'll have built an array of modules, templates, snippets, and will know exactly when to use which.
-
Based on @bernhard's suggestion, you can combine template tags with ProcessPageEdit::buildSettingsForm hook and remove any template that doesnt have a specific tag. Start by adding a specific tag to each template you need to show, say `my-template-compatible`. Then add this hook to ready.php wire()->addHookAfter('ProcessPageEdit::buildFormSettings', function (HookEvent $e) { $page = $e->object->getPage(); if ($page->template != "my-template") return; $templatesField = $e->return->children->get('id=template'); $templates = $templatesField->options; foreach ($templates as $tid => $tname) { $template = $e->templates->get($tid); if (strpos($template->tags, 'my-template-compatible') === false) $templatesField->removeOption($tid); } }); When you edit a page with `my-template` template, you'll see that non compatible templates wont show up.
-
@Robin S's ConnectPageField is perfect for this use case. You need to create another Page Reference field, say `tagged` add it to tag template. When you set up the module, when you add a tag on a page, the page will be added to tag's page reference field. Then you can just use $pages('template=tag, tagged.count>0') to find all used tags. Not really, it works on identical objects, not objects with identical values. See this for a potential solution: and this one
-
Database access denied after moving from localhost to live server
abdus replied to zkriszti's topic in General Support
Ok, after a brief Teamviewer session, it turns out that the problem is beyond PW and @zkriszti, because through neither phpMyAdmin nor adminer could we access the mysql instance. PHP cannot communicate with MySQL server at all. Either the MySQL server does not run on the localhost, but on a different server on the network, or it's not running, which I doubt, because the hosting seems to be shared, so that would mean no service for a lot of sites. Contacting the provider seems to be the best option. -
I enjoy using custom API variables in my templates. One of my usual setups is like this. // ready.php or _init.php $v = new \stdClass(); $v->class = "template--$page->template"; $v->{$page->template->name} = new \stdClass(); $v->scripts = new FilenameArray(); $wire->set('v', $v); Then at any time during template render I can add scripts using $v->scripts->add() or body class with $v->class .= "one two three", and render in my main layout. It's useful because you can always access it from templates, without needing to inject it with $files->render('template', $data) You can set it and extend with anything: a utility class, a simple array, a flag One disadvantage could be that since held in RAM all the time and injected to every TemplateFile instance, you'd need a slightly larger amount of RAM for large objects. Shameless plug: I wrote a blog post on this topic which details the points I've written above. Injection feature is for setting the current ProcessWire instance to an object. Not really needed unless you're working with multiple instances
-
Weird Error when Trying to Edit a Users Password
abdus replied to quickjeff's topic in API & Templates
It might be a namespacing issue Try adding/replacing <?php namespace ProcessWire; at the first line of the ProcessBatcher.module. If you havent disabled module compilation, then PW should compile the module and it should work even without the namespace but refreshing/retrying a few times might help as well -
After logout Fields aren't displayed on Frontpage
abdus replied to joe72joe's topic in API & Templates
When you logout, if you get 404, set the page published. If you get the page but some parts are missing, then you probably misconfigured field access settings, allow guests to view the field. -
[solved] Count # of PDFs in field and echo
abdus replied to louisstephens's topic in API & Templates
How do you access $page inside a function closure? You can't. Use wire()->page or wirePage() or page() or wire('page') -
Filter out past events when using pageReference field (front end)
abdus replied to a-ok's topic in General Support
'today' should have worked, 'now' also works. But using strtotime() is the most intuitive one