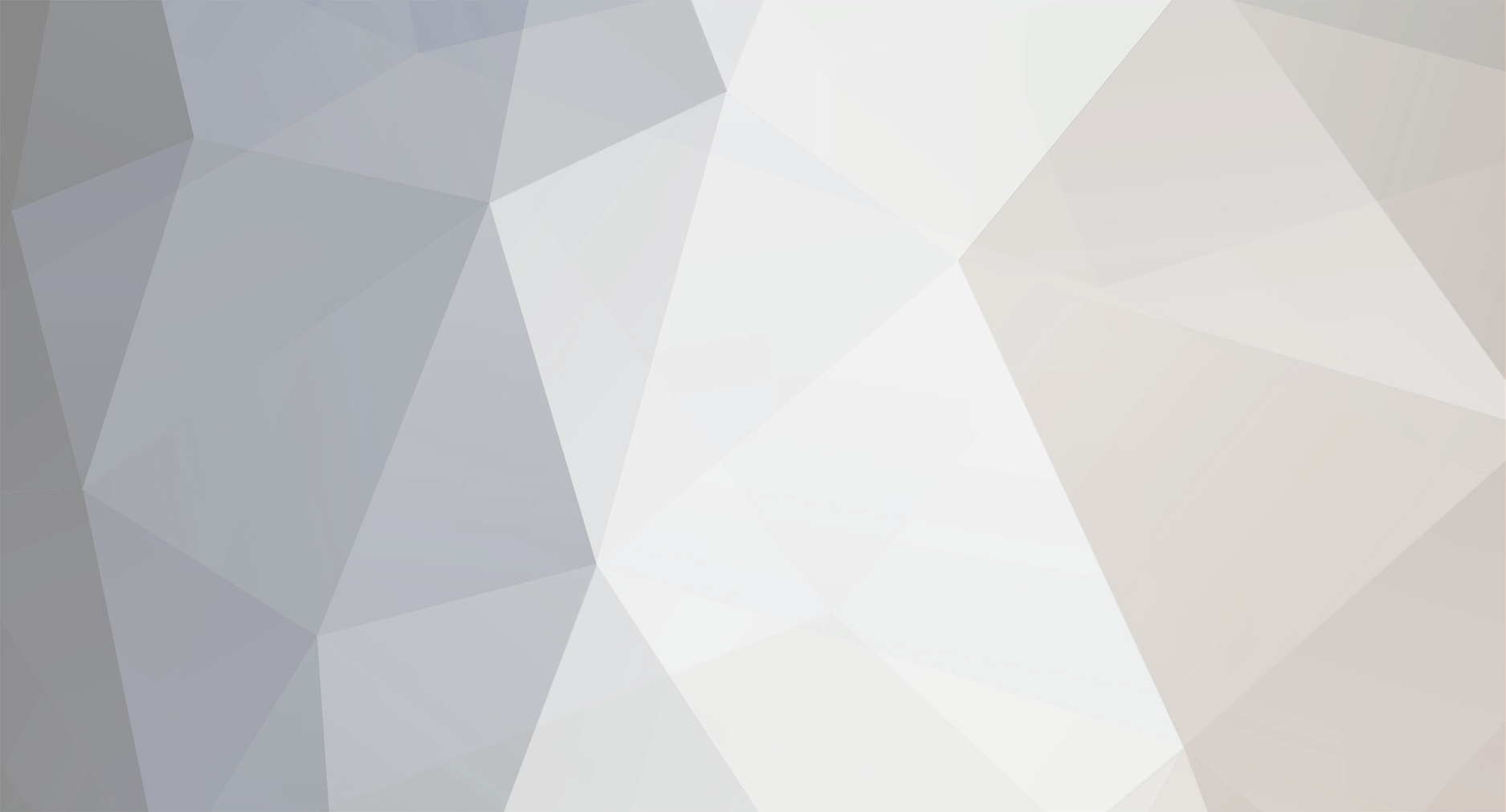
celfred
-
Posts
192 -
Joined
-
Last visited
Posts posted by celfred
-
-
Hello,
First of all, THANKS a lot for your answer which helps me better understand things. I'll go ahead and dig what you're mentionning with 'multisort'. It seems quite interesting and I have a feeling it fills a big lack in my knowledge
In the meantime, I have managed to do some stuff yesterday night which seems to do the job. In case you have a little time to read through it and see things I shouldn't do, I would be glad to have some kind of feedback on that (but as I said, I'll test your code above during the day). Roughly speaking, if I want the 'group' scoreboard, I loop through 'allPlayers' to get a list of groups and teams which I add to PageArrays. Then, I loop through all groups, get involved players and (here the thing !) I clone the group page to add it to a $uniqueGroup pageArray. And this page holds the calculated 'bonus' and 'karma'. Then, I do my thing with the 'reindex' (see code below) to try and get the logged in player's position and return all my variables to be able, then, to display a particular scoreboard (top 10, top 3 + 'surrounded' player...)
I know my explanations are hard to follow. Here's the code :
function setScoreboard($allPlayers, $player, $field) { if ($field != 'group') { $allPlayers->sort($field); } else { $allPlayers->filter("team.name!=no-team")->sort("team.name"); $allGroups = new PageArray(); $allTeams = new PageArray(); $already = []; $uniqueGroups = new PageArray(); foreach($allPlayers as $p) { $groupId = $p->team->id.$p->group->id; $p->groupId = $groupId; if (!in_array($groupId, $already)) { $allGroups->add($p->group); $allTeams->add($p->team); } array_push($already, $groupId); } foreach($allGroups as $group) { $index = 0; foreach($allTeams as $team) { $players = $allPlayers->find("group=$group, team=$team"); if ($players->count() > 0) { $newGroup = clone $group; $newGroup->team = $team; $newGroup->id = $group->id.$team->id; $newGroup->focus = 0; // Check for group bonus $newGroup->nbBonus = groupBonus($players); $newGroup->yearlyKarma = $newGroup->nbBonus*30; // Add individual karmas foreach($players as $p) { // Karma is divided by number of players in the newGroup to be fair with smaller newGroups $newGroupYearlyKarma = round($p->yearlyKarma/$players->count); (int) $newGroup->yearlyKarma = $newGroup->yearlyKarma + $newGroupYearlyKarma; $newGroup->details .= $p->title.' '; if ($p->name == $player->name) { $newGroup->focus = 1; } } $uniqueGroups->add($newGroup); $index++; } } } $allPlayers = $uniqueGroups->sort('-yearlyKarma'); } $allPlayersReindexed = new PageArray(); $allPlayersReindexed->import($allPlayers); if ($player) { if ($field != 'group') { $playerPos = $allPlayersReindexed->getItemKey($player)+1; } else { $playerGroup = $allPlayersReindexed->get("focus=1"); $playerPos = $allPlayersReindexed->getItemKey($playerGroup)+1; } } else { $playerPos = false; } if ($playerPos) { // Player is in the charts if ($playerPos <= 5) { // Good position, get Top 6 $topPlayers = $allPlayersReindexed->slice(0, 6); $prevPlayers = false; } else { $topPlayers = $allPlayersReindexed->slice(0, 3); // Get top 3 players $prevIndex = $playerPos-3; $prevPlayers = $allPlayersReindexed->slice($prevIndex, 4); } } else { // No ranking (or complete scoreboard) $topPlayers = $allPlayersReindexed->slice(0, 10); // Get Top 10 $prevPlayers = false; } return array($topPlayers, $prevPlayers, $playerPos, $allPlayersReindexed->count); }
If you want to have a look of what is the final page, you can see there (on that page, I use Ajax requests to load all scoreboards individually (and the 'group' scoreboard is actually not functionnning well), but I intended to get rid of this server load since my $allPlayers is already loaded in my head.inc, I imagined optimizing my page by simply managing my scoreboards in PHP functions.
Anyway, thanks again for your help ! And feel free to give me advice if you see horrors in my code
(but again, I'll take the time to analyze the solution in @Robin S's answer)
-
Hello,
I've been struggling for hours with this, and it is driving me crazy. I guess I completely misunderstand basic concepts, but I'm facing a wall here hence this message... Here's what I'm trying to do :
- I have a list of players in $allPlayers (pages)
- Each player has (among others) 2 pageArray fields : 'group' and 'team'.
- I'm trying to loop through all the players to build another pageArray (or WireArray or whatever...) to gather the players in groups/teams accordingly and deal with the different info I have on them and calculate a score.
- My main problem is that some players are in a different team but their group has the same name (and I need to restrict players to their own team mates).
Here's my 'code' so far (which doesn't work) :
// Build group list (for players having a team) $allPlayers->filter("team.name!=no-team")->sort("team.name"); $allGroups = new PageArray(); $uniqueGroups = []; $uGroups = new PageArray(); // This was a test, see below... $already = []; foreach($allPlayers as $p) { // Assign a groupId to each player $groupId = $p->team->id.$p->group->id; $p->groupId = $groupId; if (!in_array($groupId, $already)) { // The following lines were a test but didn't work and I DON'T UNDERSTAND WHY ? // $uG = new Page(); // $uG->of(false); // $uG->template = 'player'; // $uG->name = $groupId; // $uGroups->add($uG); // bd($uGroups->count()); // !! Keeps showing 1 ? My purpose was to be able to use $uGroups->karma, $uGroups->nbBonus... afterwards array_push($uniqueGroups, $groupId); } array_push($already, $groupId); } // Then, I loop through $uniqueGroups, get the players and calulate my score foreach($uniqueGroups as $group) { // Limit to groupId players for group scores $players = $allPlayers->find("groupId=$group"); // Check for group bonus and calculate scores [...] // I cut to simplify my post // But my other problem is storing my score : I can't use $group->karma or $group->nbBonus since $group is a ragular PHP array ? } // Then, I wanted to create a new pageArray (hence my $uGroups above) to be able to sort them with the following $uGroups->sort("-karma");
And I'm stuck... More than 5 hours today already on this, and still blocked
I've tried playing with WireData, WireArray... but I'm all lost here. You can understand why I'm posting in the 'Getting started' forum !
For further information, this part of code is embedded in a setScoreboard() function and this is the 'group' part of it.
If anyone can devote a little time to give me a clue on how to think about this, I'd greatly appreciate !
Thanks !
PS : The more I write about this, the more I think I am able to get the group members and scores (with my awkward coding), but then I am unable of simply storing this information before rendering it. Here I'm referring to my comment in my code where I say that TracyDebugger keeps showing me only 1 $uGroups page. I wish I could dynamically build a 'uniqueGroup' page with Pagefields for Group, Team, pageArray for Members, Integer fields for calculated Karma, nbBonus...... sigh......
-
Yep. I could have thought of it. Good idea !
Thanks a lot
-
Hello,
I'm facing an issue with my page fieldtype. Pages appear in the order they were created although I'd like to see them sorted in alphabetical order (by title). The pages are well-sorted in my tree, but in that particular Page fieldtype (on another page), I can't find any place where I would decide on what field I can sort them. Am I missing something here ?
Let me know if I'm not clear enough in my explanations.
Thanks in advance for a clue
-
As always in this community, a quick, straight to the point and perfect answer. Many thanks to you @kongondo
Sorry for missing it in the docs. I promise I searched for it but I was stuck in the $page part of the API and didn't see the restore()... My bad. Just to mention, I typed 'restore' in the search box (top left), but the API linked in your post above didn't appear in the results
Anyway, the answer was quite simple (just as I thought, in the PW 'philosophy') and I'm happy with that. Thanks again !
-
Hello,
I have a feeling I'm missing an easy thing here (again...). If I trash a page from API with :
mypage->trash()
Is there a simple way to restore it if needed ? I can do it in back-end, but I'd like to do it through API with something like
mypage->restore()
but this doesn't exit
So if you can give me a little help on that, I'd appreciate. Thanks in advance !
-
Thanks for your explanatory reply.
I understand the JS snippet here, but I am not able to make it work yet. The function seems not to be triggered unless I get rid of the "span[data-name="informations"] before the .pw-edit-copy (but then I imagine this works because my field is unique for my test).
I tried all sorts of changes with no luck
and I am unable to find a way to actually SEE what's going on when my field is being saved after I front-end edit it (so I can find out the correct parent to spot the exact field that's being edited).
Not sure of being clear there. Sorry if I'm not !
EDIT (before sending !) : I kept digging with my 'novice' tools and habits : I went back to my Firefox debugging console and displayed the infos of the parent while working only out of .pw-edit-copy selector and I SAW it : it was div[data-name="informations"], not <span>
As easy as it might sound to many of you, I'm glad I've managed this !
Thanks again for this answer which helps me improve.
-
2
-
-
Hello,
I can't find my way out of this. I'm trying to use the front-end editing capabilities of PW. It works quite well so far, except if the user sets an empty value. Indeed, in such a case my field just disappears until I reload my page.
Here's my code :
$out .= '<edit informations>'; if ($page->informations != '') { $out .= '<p>'.$page->informations.'</p>'; } else { $out .= '<p>No infos.</p>'; } $out .= '</edit>';
The idea is to have a paragraph showing 'No infos' if field is empty so the user can double-click on it. He/She then gets the inline editor which works perfectly well as lon as he/she sets a value. But let's say he/she decides (or by mistake) to set an empty value and save, then my paragraph disappears completely and the user must reload the page if he/she wants to edit the field again.
I wish I could add some kind of default value if field is empty. Maybe I'm misunderstanding something ?
If you have an advice, I'd appreciate
PS : I've tried all A/B/C/D front-end possibilities and read and re-read docs, but still the same issue...
-
Another nice 'lesson' from this community. Thank you very much @Robin S.
-
Hello,
Still in my 'teaching game'. Here's my problem : I 'find' all players with a request like
$allPlayers = $pages->find("template=player"); [/code Then, I limit to players belonging to the team of the logged in player with [code] $teamPlayers = $allPlayers->find("team=$loggedPlayer->team");
No problem so far. But my scoreboards rely on either :
$allPlayers->getItemKey($loggedPlayer);
or
$teamPlayers->getItemKey($loggedPlayer);
to find the logged player's position in the charts.
On the 'global' newboard with scoreboards based upon $allPlayers, everything works as expected.
BUT on my 'team' newsboard, even though I'm using $teamPlayers, the returned indexes are based upon $allPlayers. Am I clear ? In other words, I have a total of 125 players, and my logged player is 61 out of 125 regarding the number of places he freed. But in his particular team of 25 players, he sould be 15 whereas he's still 61
I'd like to reset my indexes (and start back from 0), but I can't find my way out of this...
If someone has a hint to help, I'd appreciate.
I have a second part in my worry : I had a way around it by simply making another 'raw' request :
$teamPlayers = $pages->find("team=$loggedPlayer->team");
Then my team indexes were right, but I faced another issue : Reordering my wirearray according to the scoreboard I want usually worked fine (simple sort() based upon an integer field, for example, player's coins, player's karma...) and indexes were updated BUT resorting with places.count ('places' field is a pageArray) doesn't update the indexes returned by getItemKey and my logged player is always at the position he was when I first did my initial $pages->find() query
So my way around found its limit and that's why I'm posting here, after struggling with this for a couple of hours...
Thanks in advance for the help.
-
It is an integer and I've checked the setting and intentionally set it to 'Yes' because my freeActs field had no value in some pages and I wanted those pages to be returned. In short, a player being level 1 with no previous activity should have access to items having level 1 set, enough Gold Coins (GC) and 0 previous 'free' actions...
I guess I'm not clearly understanding this yet. I'll go ahead and try to check 'No' and see what's going on, but later during the day.
Thanks for your help @Robin S
-
Hello to all,
This time, I'm stuck on an issue with a 'find' request. I have found a turnaround, but I have no idea what's going on, really, and I wish I could understand. My code is the following :
$nbEl = $player->places->count(); $items = $pages->find("template=place|item|equipment, GC<=$player->GC, level<=$player->level, freeActs<=$nbEl")->sort("template, name");
If I explain briefly : the $items should contain the possible elements for a player according to his level and GC (gold coins), and freeActs, i.e. the number of already 'bought' places.
If the 1st line $nbEl returns a number higher than 0, it 'works', I get some items. BUT if the player has not bought any place yet and then $nbEl = 0, $items is... empty whereas it should contain all elements being accessible with a 0 'freeAct' field (and there are many).
I have tried to hard-code 'freeActs<=0', I get no items. 'freeActs<=1', I get some nice results' AND THEN, 'freeActs=0' and it works as expected
So here's my 'workaround' :
$nbEl = $player->places->count()+1; $items = $pages->find("template=place|item|equipment, GC<=$player->GC, level<=$player->level, freeActs<$nbEl")->sort("template, name");
Note the 'freeActs<$nbEl' instead of 'freeActs<=$nbEl' and the '+1' for $nbEl so not to start at 0... Then, it works as expected !?!?
Does someone have an explanation for this ?
Thanks in advance if you do. And don't hesitate asking me for more details/explanations if you need.
-
@kongondo Sorry for being late on that... Thanks for your reply. Somehow, your answer is comforting since things should work the way I was understanding them. Now I'll try and find out what's going on in my code.
Good point about my wrong variable name. I wasn't even aware of that... Shame on me...
-
Thanks. But is that new in some way ? Or was it always like that but I didn't notice ?
Just making sure I'm being understood : the equipment is added to the player's page, BUT it stays in the equipment list even though I reload the page and empty the cache. I thought it wouldn't stay since I didn't save the page.
-
Hello,
Just a simple (I think) question which is in the title of my post.
Roughly speaking : here's my code (made-up because my real function is so long... I don't want to post it all here)
function updateScore($player, $task, $real = true) { [...] $player->score = $player->score+$task->score; if ($task->name == 'new-equipment') { $new-eq = $pages->get("name=sword"); $player->equipment->add($new-eq); } if ($real == true) { $player->save(); } }
Everything works fine when I call updateScore($player, $task, true), but if I call updateScore($player, $task, false), scores are untouched, but the equipment gets added !
It used to work fine on PW2.7 but my update to PW 3.0.62 seems to have broken this... Is there a simple explanation ? I keep reading my code over and over and this is driving me crazy...
Thanks !
-
Here's what I think : I had an 'old' processwire directory which I downloaded through Git and which I thought was up-to-date with master (not dev branch) so I really imagined I was with 3.0.62. (I'm sure I did a git pull but I guess not...)
And today I added PW version in my footer and saw 3.0.36 and imagined I forgot about the version number _ must have been 3.0.32 and they've already made some changes and now it's 3.0.36... Anyway, you see how good I am with Git and some stuff
So I'm really sorry about all these issues which shouldn't have happened if I had done things correctly. I'll update for real (!) and see how it goes.
Thanks again for your help and time. I appreciated a lot !
And about my Tracy 'theme' : I just use my computer 'negative' mode because I have eyesight problems and I feel better this way. So no leaking, don't worry
EDIT : 3.0.62 installed : I can confirm, there is no more a bug with the 2 forms of 'find' statements.
-
1
-
-
-
It returns 'NULL'. How does that sound ?
-
Well, I've checked my localhost version and it gives 5.7.19.
Anyway, I've changed my line taking the 'sort' out of my 'find' string and it works (actually, I believe my 'sort' command is not really useful on my page any longer so it's not really an issue for me any longer). I've taken care of most of my errors (I think) and it seems to work fine.
I went ahead and made the changes on my live site. It looks ok. I'll test tomorrow when actually saving data 'for real', but on my localhost it works, so that's cool ! I'm running on PW 3.0.36 now
Thanks a lot for your help !
If anything still matters to you and you suspect a weird behavior on PW side and you want to dig this a little, don't hesitate asking me for further testing or information.
-
Hello,
I'm back to it and it's not easy
203 PDO queries in my Tracydebugger ! That sounds like a lot to me... I have tried to isolate the 'responsible' one and here it is (I think) :
SELECT pages.id,pages.parent_id,pages.templates_id FROM `pages` JOIN field_team AS field_team ON field_team.pages_id=pages.id AND ((((field_team.data='85366') ) )) LEFT JOIN field_group AS _sort_group ON _sort_group.pages_id=pages.id LEFT JOIN pages AS _sort_page_group_name ON _sort_group.data=_sort_page_group_name.id WHERE (pages.templates_id=43) AND (pages.status<1024) GROUP BY pages.id ORDER BY _sort_page_group_name.name
This corresponds to the following statement :
$allPlayers = $pages->find("template=player, team=$team, sort=group");
Answering your question : the 'group' field is indeed a 'Page' type. It used to be a 'Text' type so I tried and changed my find query replacing 'sort=group' by 'sort=group.name' and the error is still there. Here's the resulting PDO query in Tracydebugger :
SELECT pages.id,pages.parent_id,pages.templates_id FROM `pages` JOIN field_team AS field_team ON field_team.pages_id=pages.id AND ((((field_team.data='85366') ) )) LEFT JOIN field_group AS _sort_group_name ON _sort_group_name.pages_id=pages.id LEFT JOIN pages AS _sort_page_group_name ON _sort_group_name.data=_sort_page_group_name.id WHERE (pages.templates_id=43) AND (pages.status<1024) GROUP BY pages.id ORDER BY _sort_page_group_name.name
As I said earlier, if I change the syntax to :
$allPlayers = $pages->find("template=player, team=$team")->sort("group.name");
The PDO query shows :
SELECT pages.id,pages.parent_id,pages.templates_id FROM `pages` JOIN field_team AS field_team ON field_team.pages_id=pages.id AND ((((field_team.data='85366') ) )) WHERE (pages.templates_id=43) AND (pages.status<1024) GROUP BY pages.id
and everything works as expected.
I've tried my best to be helpful. I hope I've managed to isolate the correct lines and that you can understand something out of it. (and that I'm not responsible for taking this time from you... by responsible, I mean that I did something wrong that caused the problem, which can be a possibility)
Sidenote : Trying to isolate the query, I took almost everything out of my page (no more find/get statement except that one, no more head.inc, foot.inc....) and I still have either 41 or 38 (depending on reload) PDO queries on my page). I would imagine that's PW 'doing his job'. Is that so ?
-
I have the TracyDebugger installed and I'll try to do what you're asking me with pleasure, but I have to stop right now (time to eat at home, daughters hungry...
) I'll do that tomorrow and keep you updated about my progress.
And I agree with your advice about installing modules directly from within ProcessWire. It is indeed quite simple
-
1
-
-
Last thing for the moment while trying to update to PW 3.x : in backend, my modulemanager page tries to read a modulemanager.cache file in files/assets/cache which doesn't exist hence error messages :
Warning: fread(): Length parameter must be greater than 0 in /home/celfred/PlanetAlert/site/modules/ModulesManager/ModulesManager.module on line 812
ProcessWire: ModulesManager: cannot read cache file /home/celfred/PlanetAlert/site/assets/cache/ModuleManager.cache
(with debug true)
-
Here's what I did :
- Restarted mysql to be back with the initial fatal error.
- Found out that commenting out the line : $allPlayers = $pages->find("template=player, team=$team, sort=group"); got rid of the error
-
Replaced the line with : $allPlayers = $pages->find("template=player, team=$team")->sort("group"); and... It works
That's indeed a cleaner solution better than changing my mysql settings. I guess the 'bug' was on my side with the 'sort' command included in my 'find' query. I have to revise the PW doc to see where I saw that syntax or if I made it up without noticing that it never worked (which is possible !).
So thanks for pointing this out to me !
-
EDIT : As a follow-up, still digging on my updating issues : I have found that post : https://stackoverflow.com/questions/23921117/disable-only-full-group-by#36033983
Along with the namespace line on my particular page, it works
EDIT #2 : Sorry ! I validated another post instead of editing the first one.
Filtering / Type / Array issues
in Getting Started
Posted
Still trying to understand things better. I've been looking at @Robin S's answer and I'm facing another issue. To set my nbBonus for each group, I had this function :
function groupBonus($players) { $nbBonus = 0; // Sort players by nb of places $players->sort('places.count'); // Get min/max nb of places in the group $min = $players->first()->places->count; $max = $players->last()->places->count; if ($min == 0) { // 1 player has 0 places, so NO bonus possible return 0; } else { // No player has 0 places, let's check if they all have 1,2,3... places for ($i=1; $i<=$min; $i++) { $nbPlaces = $players->find("places.count>=$i")->count; if ($nbPlaces == $players->count) { $nbBonus++; } } } return $nbBonus; }
So my parameter $players was a PageArray() I had thanks to a 'find' request. But now, it is a regular PHP array and this throws an error because I'm trying to use lines such as $players->sort('places.count')...
All in all, I was trying to rework my function to work on regular PHP arrays, but then I have other headaches because I use many PW ways afterwards and I eventually wondered if I could simply turn my $players regular PHP array into a pageArray() again ?
And eventually, I wonder what you feel seems to be the best way for me ? Would it be preferable to work on regular PHP arrays all through my functions or should I 'convert' as soon as possible (if possible) so I can use PW way (or what I understand being PW way...)
And if you can give me a hint on what I should read more about (at this point) concerning my programming skills, I'd appreciate as well because I see that my skills are getting too low for what I want to do on my project
Thanks !