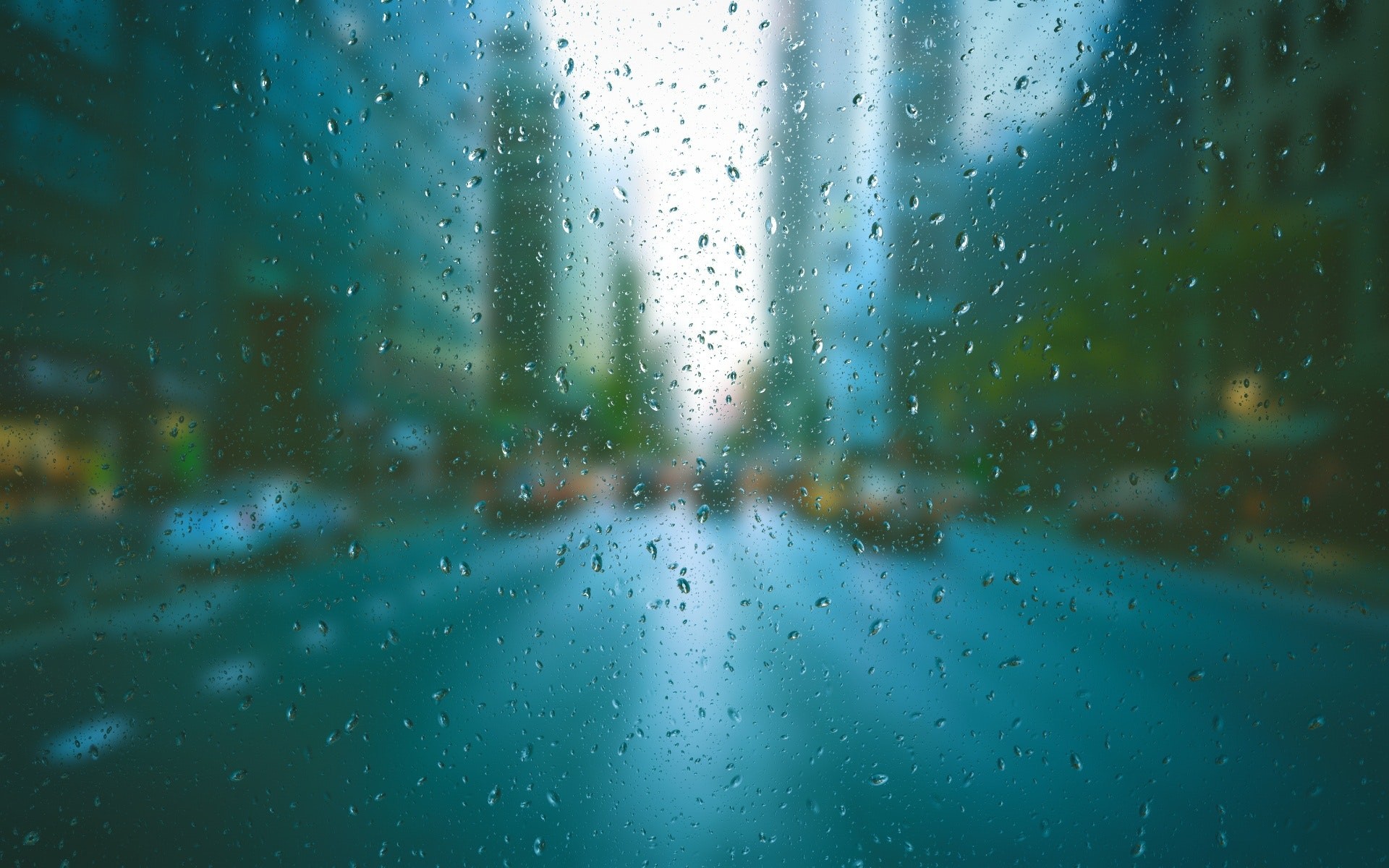
Dean
-
Posts
60 -
Joined
-
Last visited
Posts posted by Dean
-
-
I've realised the issue is that when the user page is created, if I filled out the form as dave@gmail.com, the page is create as dave-gmail.com. And so I need to log in as dave-gmail.com, not dave@gmail.com.
So I'm guessing I need to set the user page title as the person's name when I'm creating it? Let me know what you think @Jan Romero ? -
After setting up a new user template using this guide: https://processwire.com/blog/posts/processwire-core-updates-2.5.14/#multiple-templates-or-parents-for-users, the pages are created fine but I can't log in as the newly created user.
I did notice that my new template doesn't have a 'name' field like the users in admin/access/users. Is this the field that is checked when logging in? Here is my code that I am using to create the page when someone registers:
$np = new Page(); // create new page object $np->template = $templates->get("user-hunt"); $np->parent = $pages->get("/members/"); // Match up the sanitized inputs we just got with the template fields $np->of(false); $np->name = $hunt_name; $np->title = $hunt_name; $np->member_hunt_name = $hunt_name; $np->member_hunt_contact_name = $contactname; $np->member_hunt_position = $position; $np->member_hunt_address = $address; $np->member_hunt_postcode = $postcode; $np->member_hunt_telephone = $telephone; $np->member_hunt_country = $country; $np->email = $email_address; $np->pass = $password; // Save the page $np->save(); $np->of(true);
Also, when it creates the page, it's putting the title as the created date/time for some reason. ?
-
Thanks once more @Jan Romero. It is now creating a user under /members/.
Just to clear things up, is what it has created under /members/ a page or a user? Or both? ?
-
Sorry, one last question. How can I now create my new user with the new template and new parent? It doesn't seem to like this:
wire()->addHookBefore('LoginRegister::createdUser', function($event) { $u = $event->arguments[0]; $u->parent = 1083; // set new parent $u->addRole('hunt'); $u->save(); });
-
Brilliant! That was it. Thank you so much @Jan Romero.
-
Thank you so much @elabx and @flydev ??. I thought that was it, that I wasn't passing the variable into the function.
However, it is now somehow giving the user the default value returned from getRole(), rather than the value it should give. And yet, if I echo the value returned from getRole() before and after executing loginRegister, it is correct! ?
$myRole = getRole($input->post('register_member_role')); echo $input->post('register_member_role').' gives '.$myRole; // value is correct here wire()->addHookBefore('LoginRegister::createdUser', function($event) use($myRole) { $u = $event->arguments[0]; $u->addRole($myRole); $u->save(); }); echo $loginRegister->execute(); die($myRole); // value is correct here
-
I don't seem to be able to add a role to a user when they register.
I have a function to return the role as text based on the option that was chosen on the register form. I have checked that this function is returning the correct value by echoing it to the page. If I pass that text into the addRole function (as $myRole) it doesn't work. If I hard code the exact same text into addRole it does work.
Do I need to change my getRole function to return the text in a different format?
function getRole($role) { // return name of role based on id from register_member_role switch ($role) { case 1: return "type1"; break; case 2: return "type2"; break; case 3: return "type3"; break; default: return "type1"; } }
$loginRegister = $modules->get('LoginRegister'); $myRole = getRole($input->post('register_member_role')); wire()->addHookBefore('LoginRegister::createdUser', function($event) { $u = $event->arguments[0]; $u->addRole($myRole); $u->save(); }); echo $loginRegister->execute();
-
-
Thank you @Jan Romero. For some reason, when I'm adding a new user now, I get '/processwire/access/users/' as the only option for the parent. Have I forgotten something?
-
Hi
I'm creating a site where people can vote on dogs.
To start with, I need the dog owners to register and then upload text, images, and videos about their dog. What I thought I could do is to create a page when the user registers. The page would be unique by using the member ID. So I would end up with:
Home
- Members
-1001
-1021
-1025
Then each member page would have 1 or more children which would be the dogs that they have added.
I just wanted to ask if that is a good way of doing things or if there's maybe a much simpler way of doing this.
Thanks in advance for any advice.
-
Sorry, but I have added several fields to the user template.
I am then using:
$loginRegister = $modules->get('LoginRegister'); echo $loginRegister->execute();
But still I only get the email address and 2 x set password boxes. Have I forgotten something?
-
Thanks Sephiroth. Although I think that is too advanced for me at this stage. It looks very impressive though. I will try to do it a less fancy way.
-
Thank-you. I did wonder whether I could keep it all separate so that I didn't have to give the public access to /processwire.
So if I keep my member pages part of the front-end and then create pages based on what they add. Should I use the person's user id to keep the directory structure unique? E.g., members/46/my-page/.
-
Dear ProcessWire community
Before I jump right into this new project of mine and then realise it's not going to work, I'd be very grateful if I could get some advice on the best way to achieve this.
My client wants people to be able to pay a small amount when registering to become a member. To help with this I have just bought Ryan's FormBuilder with Stripe payments module.
We then need the member to be able to log in and upload a few images (which I'll make a gallery out of) and possibly a short video.
What I'm hoping to do then is to create a user/role which will allow the member to log in to ProcessWire and have access to just a certain area to allow them to upload their content.
So I wanted to ask if that is a) possible, and b) the best way to go about it.
I have used ProcessWire before, but it was a few years ago so any help and advice anyone can give would be greatly appreciated. -
So easy, thanks!
-
Hi, I currently have this code:
$navigation = $page->rootParent->navigation
if(count($navigation) > 0)
{
foreach($navigation as $p)
{
$children = $p->children;
if(count($children) > 0)
{
// accordion header
}
else
{
// simple link
}
}
}
for outputting my navigation links, which works fine. But, for one section (news), I don't want the subpages (articles) to appear in the navigation. I have edited the code so that
if(count($children) > 0)
becomes
if(count($children) > 0 && $p->title != 'News')
so that news articles are excluded. But I think this is not a good way to do it. Can anyone share the normal/best way to exclude pages like this?
-
Thanks Craig, Raymond. I will try these options and see which works best for me.
-
Craig, thanks for this. I think I understand what you mean, but would this only allow me to add one 'other' link to my page? Or I am not getting it?
Ideally, I would need to be able to have a menu like:
<child page of this section>
<child page of this section>
<link to another page in the site>
<child page of this section>
<link to another page in the site>
<child page of this section>
-
Hi all
I have successfully built my very first navigation menu. It works by looping through the pages within a section and echoing the page title and url. The problem is, sometimes I will need a navigation link to take me to a page within a different section to the current one, and I have no idea how I could achieve this. Has anyone ever had to do this themselves, or have an idea how it could be achieved?
New User Template Not Allowing Log In
in General Support
Posted
Thanks @Jan Romero, I decided to abandon the LoginRegsiter module as I needed to send emails when people logged in and other stuff and I thought it would be easier to start from scratch rather than try to amend something I hadn't written.
After reading lots of other forum posts, my code has changed to this now:
if($input->post->hunt_name) { // sanitize input field values $user = $sanitizer->userName($input->post->hunt_name); $email = $sanitizer->email($input->post->email_address); $password = $input->post->password; // create user $registerUser = createUser($user, $email, $password); $registerUser->of(false); $registerUser->member_hunt_name = $hunt_name; $registerUser->member_hunt_contact_name = $contactname; $registerUser->member_hunt_position = $position; $registerUser->member_hunt_address = $address; $registerUser->member_hunt_postcode = $postcode; $registerUser->member_hunt_telephone = $telephone; $registerUser->member_hunt_country = $country; // save $registerUser->save(); $registerUser->of(true); } function createUser($user, $email, $password) { // create user $u = new User(); // set template and parent $u->template = $templates->get("user-hunt"); $u->parent = $pages->get("/members/hunts/"); // set name $u->name = $user; // set email $u->email = $email; //set password $u->pass = $password; // assign role $u->addRole("hunt"); // save new member $u->save(); return $u; }
This is creating a page in /members as 0.78273300-1590946171. ?