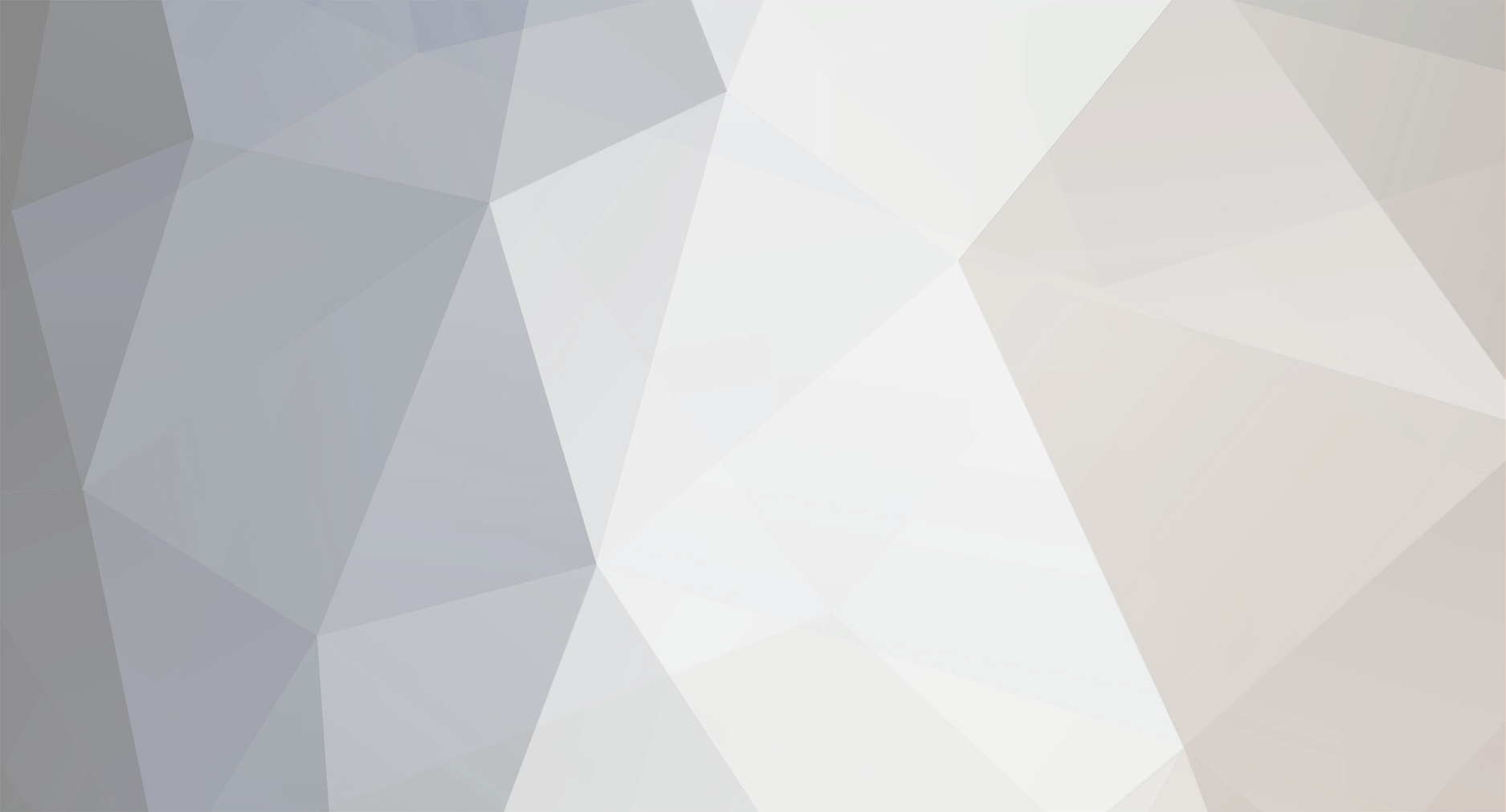
taotoo
Members-
Posts
71 -
Joined
Everything posted by taotoo
-
Efficiently finding next/prev page, but not by traversing the page tree
taotoo replied to taotoo's topic in General Support
You've nailed it, thank you very much Kongondo! -
Efficiently finding next/prev page, but not by traversing the page tree
taotoo replied to taotoo's topic in General Support
I've made the code more concise, but not sure how to rearrange it to avoid the error message on each click of the Next link subsequent to the first. <!--Discover the current exhibition from the URL parameter--> <?php $url_exhibition = $input->get->text('exhibition'); ?> <!--Find all works in the current exhibition so we can find the current $key--> <?php $from_exhibition = $pages->get("template=exhibition, name=$url_exhibition"); ?> <?php foreach ($from_exhibition->exhibition_work as $key => $from_exhibition_work): ?> <!--Set $currentkey as the current work key--> <?php if ($from_exhibition_work->title == $page->title): ?> <?php $currentkey = $key; ?> <?php endif; ?> <!--Add +1 to current work key (so we can use it for the 'next' arrow)--> #59 <?php if ($key == $currentkey +1): ?> <!--Make a next arrow link--> <a href="../<?php echo $from_exhibition_work->title . '/' . '?exhibition=' . $url_exhibition ?>">NEXT</a> <?php endif; ?> <?php endforeach; ?> -
I have a page tree structure like this: Exhibition - Exhibition 1 - Exhibition 2 - Exhibition 3 ... Work - Work 1 - Work 2 - Work 3 - Work 4 - Work 5 - Work 6 ... Works are assigned to Exhibitions via two page reference fields (using Connect Page Fields): exhibition_work in the Exhibition template work_exhibition in the Work template A given exhibition will include some of the Works, but not all. On the front end I have Exhibition pages (using the Exhibition template), each of which shows their Works in a grid (sorted manually with the page reference field UI). When a Work is clicked on it takes you to the Work page (Work template) for that particular Work. This is all working fine. *The issue* On the individual Work pages, I want to have prev/next arrows so that the user can navigate to adjacent Works. The arrows can't just link to the prev/next page in the tree, as that page may not appear in the relevant exhibition. I've got it working by using a URL parameter, and copying the relevant query from the Exhibition template, pasting it twice into the Work template and then fiddling with it (code below). Is there a more efficient way of doing this? (I've also tried the code from this post (thank you @Robin S) but I couldn't get the sort order to reflect the Page Reference field's manual order.) <!--Discover the current exhibition from the URL parameter--> <?php $url_exhibition = $input->get->text('exhibition'); ?> <!--Find all works in the current exhibition so we can find the current $key--> <?php foreach ($pages->find("template=exhibition, name=$url_exhibition") as $from_exhibition): ?> <?php foreach ($from_exhibition->exhibition_work as $key => $from_exhibition_work): ?> <!--Set $currentkey as the current work key--> <?php if ($from_exhibition_work->title == $page->title): ?> <?php $currentkey = $key; ?> <?php endif; ?> <?php endforeach; ?> <?php endforeach; ?> <!--Add +1 to current work key (so we can use it for the 'next' arrow)--> <?php $currentkeyplusone = $currentkey +1; ?> <!--Find all works in the current exhibition (again!) so we can isolate the next work for the forward arrow--> <?php foreach ($pages->find("template=exhibition, name=$url_exhibition") as $from_exhibition): ?> <?php foreach ($from_exhibition->exhibition_work as $key => $from_exhibition_work): ?> <!--Make a next arrow link--> <?php if ($key == $currentkeyplusone): ?> <a href="../<?php echo $from_exhibition_work->title . '/' . '?exhibition=exhibition1' ?>">NEXT</a> <?php endif; ?> <?php endforeach; ?> <?php endforeach; ?>
-
I have a repeater that outputs a list of names, and a form so that people can add their name to the list: <?php echo $page->$title; ?> <?php foreach($page->repeater as $repeat): ?> <?php echo $repeat->text; ?> <?php endforeach; ?> <form action="/" method="post"> <label for="repeat">Name:</label> <input type="text" name="repeat"> <button type="submit">Submit</button> </form> e.g. Monday Ann Ben Charlie Name: [ ] [Submit] At the top of the page I have this to process the form: <?php if ($input->post->repeat) { $repeat = $page->repeater->getNew(); $page->of(false); $repeat->text = $input->post->repeat; $page->repeater->add($repeat); $page->save(); } ?> Now I want to have the same, but for every day of the week, all on one page, like this: Monday Ann Ben Charlie Name: [ ] [Submit] Tuesday Darren Ben Fred Name: [ ] [Submit] ... So I've nested a repeater like this: <?php foreach($page->repeater as $repeat): ?> <?php echo $repeat->text; ?> <?php foreach($repeat->repeater2 as $repeat2): ?> <?php echo $repeat2->text; ?> <?php endforeach; ?> <form action="/" method="post"> <label for="repeat2">Name:</label> <input type="text" name="repeat2"> <button type="submit">Submit</button> </form> <?php endforeach; ?> And updated the other code like this: <?php if ($input->post->repeat2) { $repeat2 = $page->repeater->repeater2->getNew(); $page->of(false); $repeat2->text = $input->post->repeat2; $page->repeater->repeater2->add($repeat2); $page->save(); } ?> But when I try to add a new name using the form, I get this error: Call to a member function getNew() on null ..on this line: $repeat2 = $page->repeater->repeater2->getNew(); I guess I need to tell "getNew" which of the nested repeaters I want the new row to be appended to. I thought maybe I could add a second field to the form, something like: <input type="text" name="repeaterRowID" value="<?php echo $repeat; ?>"> (The outer repeater row ID seems to be similar to the inner repeater ID) And then change the "getNew" line to something like: $repeat2 = $pages->repeater("id=($input->post->repeaterRowID)")->repeater2->getNew(); But can't figure it out...
-
In the end I went back to trying to reorder the pagination pages, and I only needed to add Jan's code like this: <?php $totalPages = ceil($posts->getTotal() / $posts->getLimit()); ?> <script> var nextPages = [<?php for ($i = $input->pageNum+1; $i <= $totalPages; $i++) { echo "'page{$i}',"; } for ($i = 1; $i <= $input->pageNum -1; $i++) { echo "'page{$i}',";}?>]; </script> And then set infinite scroll's options like this: <script> $('.infinite-container').infiniteScroll({ path: function() { if (this.loadCount < <?php echo $totalPages; ?>) { return nextPages[this.loadCount]; } } }); </script> So thank you Jan!
-
Unfortunately it appears that reordering the pagination pages won't work as the infinite scroll script only ever reads the initial link, and assumes that all further page numbers will increase sequentially. So I'm trying to slice the actual pages instead: <!--Get all dogs--> <?php $data = $pages->find("parent.template=adopt, sort=sort"); ?> <!--Get current pagination page dogs and all later pagination page(s) dogs--> <?php $data1 = $data->slice((($input->pageNum * 4) - 4),100); ?> <!--Get previous pagination page(s) dogs--> <?php $data2 = $data->slice(0, (($input->pageNum * 4) - 4)); ?> <!--Merge and set limit for pagination--> <?php $posts = $pages->find("id=$data1|$data2, limit=4"); ?> This seems to work, however while the two groups come in the order that I want, the manual order of individual items isn't respected (it's using date created or similar instead), e.g: 23 21 24 22 4 1 3 2 If I add "sort=sort" to the last line, the manual order of individual items is respected, but the two groups unfortunately also respect that order, e.g. 1 2 3 4 21 22 23 24 What I actually want is: 21 22 23 24 1 2 3 4
-
I think ideally the current pagination page would appear first, so while on page 4 it would look like < 456123 >, with "4" being non-clickable. This code looks great, thank you! The infinite scroll that I'm using gets the next page from the ">" link rather than from the page number links themselves. How would I create a next page link that reflects the new order? I'm looking at your code but I don't have any experience of for loops, and limited experience of the ++ thing! Edit: I added this line before the first for loop to add in the current page: echo "<li>{$input->pageNum}</li>"; And added "- 1" into the below to end it one page earlier: for ($i = 1; $i <= $input->pageNum - 1; $i++) But I'm struggling to create the next page link that infinite scroll needs.
-
Thank you. I'd like to apply your first line of code to the pagination links, but not sure how. Here are (hopefully) the relevant parts of the code: <?php $posts = $pages->find("parent.template=adopt, limit=4, sort=sort"); ?> <?php $pagination = $posts->renderPager(array( 'nextLinkMarkup' => "<a class='page-link next' href='{url}#more-dogs'><span>{out}</span></a>" ));?> <div class="row infinite-container" data-infinite-scroll='{ "path": ".next"}'> <?php foreach ($posts as $post): ?> <div class="col-lg-3 infinite-post"> [ ] </div> <?php endforeach; ?> <?php echo $pagination; ?>
-
Is it possible to change the pagination order, from: < 123456 > to: < 561234 > The exact number which the pagination would start on would need to be the current pagination page (pageNum, I believe). It's actually the 'next page' arrow at the end that I need to modify, but it's easier to show what I'm getting at with the page numbers. Longer version: I have product pages which show a single product. Beneath the single product, I want to show a grid of other products. I want to show all products that follow the current product first, then maybe have a horizontal line and show all the products previous to the current one. This is so that the visitor doesn't have to scroll past a bunch of products that they've probably already scrolled past shortly before, while still allowing them to see all the products if they want to. The grid will use infinite scroll, which does it's thing based on the link on the 'next page' pagination item. edit: the products are manually sorted in the backend.
-
I'm uploading 8 images simultaneously, averaging about 4mb each, with dimensions between 3,000 and 6,000 pixels. They're being downsized to four different variations, including the default variation. Php 7.0 in Xammp, and 7.2 on server (the issue is present in both environments). Edge takes 42 seconds to do this. Firefox takes 35 seconds. Safari (in a virtual machine) 1 minute 8 seconds. Chrome takes over 5 minutes, sometimes while having to click through Chrome "page unresponsive" messages. I've disabled all Chrome extensions. Chrome dev tools:
-
*Now fixed, please ignore* ------------------------------ My site has English and Thai language (not sure if relevant). The live version of the site works fine, but in my local version (using XAMPP) I cannot change the admin password as the password confirmation field is greyed out (similarly I can't create a new user). Chrome's console shows this: Here's line 1808: The console also shows this: Both the local and live databases appear to have collation generally set to utf8_general_ci, and the field_pass columns set to ascii_general_ci. Maybe the below is the issue? Local: Live:
-
I've been staring at this for a while and finally figured out why it works - very clever!
-
That works great - I'll now go through your code and attempt to understand what it's doing - thank you!
-
Thank you Robin - I could have been clearer, both page reference fields are on the Work template: work - work_exhibition - work_artist
-
I have three types of content, with two page reference fields linking them. work / \ work_exhibition work_artist / \ exhibition artist On the Exhibition template, I want to show all artists that appear in an exhibition, and beneath each artist all works that are both by them and in the exhibition. I have the following code: <?php foreach ($pages->find("template=work, work_exhibition=$page->path") as $workIds):?> <?php foreach ($pages->find("template=artist, title={$workIds->work_artist->title}") as $artist):?> <strong><?= $artist->title; ?></strong><br> <?= $workIds->title; ?><br><br> <?php endforeach; ?> <?php endforeach; ?> Which is giving me (e.g.): Artist 1 Work Artist 1 Work Artist 2 Work I'd like it to instead show: Artist 1 Work Work Artist 2 Work I've tried using "->unique()" but wasn't sure where to put it. Or maybe I need to go about this some other way. Any pointers appreciated.
-
Interestingly, if I remove ARTIST from the the pages.find line, and instead put it in a conditional, it then works fine. <!--Show artists that are related to current exhibition--> {% set ARTIST = page.exhibition_artist %} {% for artist in artist %} {{ artist.title }} <!--Show works that are related to current exhibition and belong to a given artist--> {% set work = pages.find("template=work, work_exhibition=[page.path]") %} {% if work.work_artist==ARTIST %} {{ work.title }} {% endif %} {% endfor %}
-
Thanks for this - looks neater than what I came up with - I'm sure I can learn from it.
-
Thanks - unfortunately it's still not working though. I actually managed to do it in PHP, but I thought Twig might be easier to learn. This works fine: <?php foreach($page->exhibition_artist as $a) { echo "$a->title"; /*Get works whose work_exhibition field points to the current last segment and work_artist field points to the given artist*/ $wor = $pages->find("template=work, work_exhibition=[{$page->path}], work_artist=[$a]"); /*Render works*/ foreach ($wor as $w) { foreach($w->work_image as $image) { echo " <img src='$image->url' width='200'>"; } /*Render artists*/ echo "{$w->title}"; } } ?>
-
I'm a beginner and was wondering why the code below isn't working. The issue is where ARTIST (capitalised) appears in the code for the second time. I want it to take the value from the first mention of ARTIST, but it's not doing anything. If I replace the second instance with the name of an artist, then it displays as expected. Hopefully I'm making a basic error! <!--Show artists that are related to current exhibition--> {% set ARTIST = page.exhibition_artist %} {% for artist in artist %} {{ artist.title }} <!--Show works that are related to current exhibition and belong to a given artist--> {% set work = pages.find("template=work, work_exhibition=[page.path], work_artist=[ARTIST]") %} {% for work in work %} {{ work.title }} {% endfor %} {% endfor %}